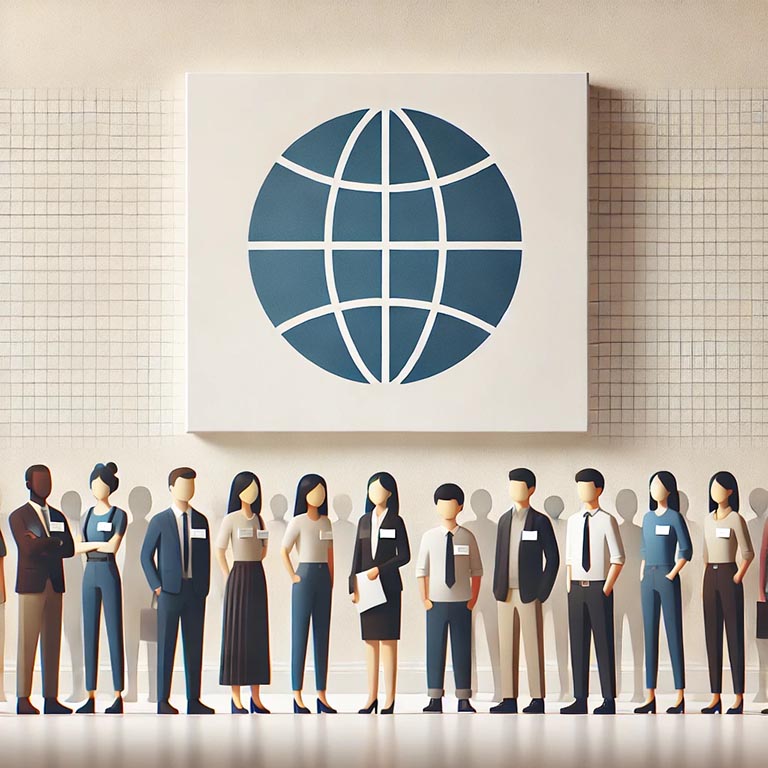
By: Ron Miller | Published: December 17, 2024
Logging Web Session IDs in JavaScript
A web session ID acts like a name tag that follows a user around while they’re on your site, right up until the browser tab is closed. Logging that ID with every log makes it easier to figure out problems. If there's an issue, you can filter logs for that specific session or perform aggregations based on multiple sessions, like “the average amount of time spent on site” or “the count of unique sessions this week.”
Don’t confuse a web session ID with OpenTelemetry’s trace ID and span ID. The latter relate to the lifespan of a request as it travels between multiple services, while a web session relates to the client-side lifespan that can initialize many requests.
Creating and saving a web session ID
The lifespan of a session ID starts when the user opens the site and ends when the user closes the tab. Conveniently, the browser API contains a storage object with just that lifespan: sessionStorage
. Here’s a simple implementation for saving a session ID:
public getSessionId() {
let session = sessionStorage.getItem('sessionId');
if (session) {
return session;
}
session = this.generateSessionID();
sessionStorage.setItem('sessionId', session);
return session;
}
This is a lazy implementation that generates a session ID the first time this function is called (which doesn’t necessarily happen at the actual session start). Once generated, the session ID is saved in sessionStorage
, and on subsequent calls, the saved ID will be returned.
For the actual session generation, a simple random GUID will do. Here’s one the way of doing this:
private generateSessionID() {
return 'xxxxxxxx-xxxx-4xxx-yxxx-xxxxxxxxxxxx'.replace(/[xy]/g, function (c) {
const r = (Math.random() * 16) | 0;
const v = c === 'x' ? r : (r & 0x3 | 0x8);
return v.toString(16);
});
}
Logging your web session ID
You probably wouldn’t want to call getSessionID()
in every log statement.
// we don't want this
log.info('something happened', getSessionID());
// rather, we want this
log.info('something happened');
There are several ways of getting the session ID added automatically. Maybe the easiest way is to use a wrapper class, like this (in TypeScript):
export class Logger {
public debug(message: string): void {
this.log(LogLevel.Debug, message);
}
public info(message: string): void {
this.log(LogLevel.Info, message);
}
public warn(message: string): void {
this.log(LogLevel.Warn, message);
}
public error(message: string): void {
this.log(LogLevel.Error, message);
}
// --------------- main function:
private log(message: string): void {
const sessionID = getSessionID();
// ....
}
}
Another way would be to use “Enrich” functions if you’re using a logging library that allows it.
Using Web Session IDs on the Backend
Once you have your web session IDs on your logging server, you can gain a lot of insights. Here are some ideas:
- Debugging: When you have your session ID, you can filter logs from that session and see everything that happened up to the point of the issue you’re investigating.
- Expose the web session ID to the user: Letting your users copy the session ID and attach it to a support ticket is an easy way to always find the right logs.
- Per-session statistics: A session is a very interesting logical unit. You might want to know the percentage of sessions where users converted, how many errors occurred in a session at the 95th percentile, or how long users spend on your site on average. That’s pretty important information. A lot of sites use Google Analytics for that, but there are myriad reasons why that’s not an option.
- Tracking user behavior: There are many insights you can gather from knowing what actions happened during a session. For example, “In how many sessions did the user press button A?” Or “In how many sessions where the user pressed button A did they also press button B?” Just note that many log management vendors don’t support answering such complex questions. For that, you’ll need a logging solution that supports advanced analytics, like Obics.